JavaScript testing can be daunting, especially when working on complex projects that require a significant amount of code. However, by leveraging the right tools and frameworks, you can write efficient tests that not only catch bugs but also streamline the development process. In this article, we’ll be discussing ten tips for writing efficient JavaScript tests using frameworks, with a particular focus on Jest.
1. Use a Testing Framework
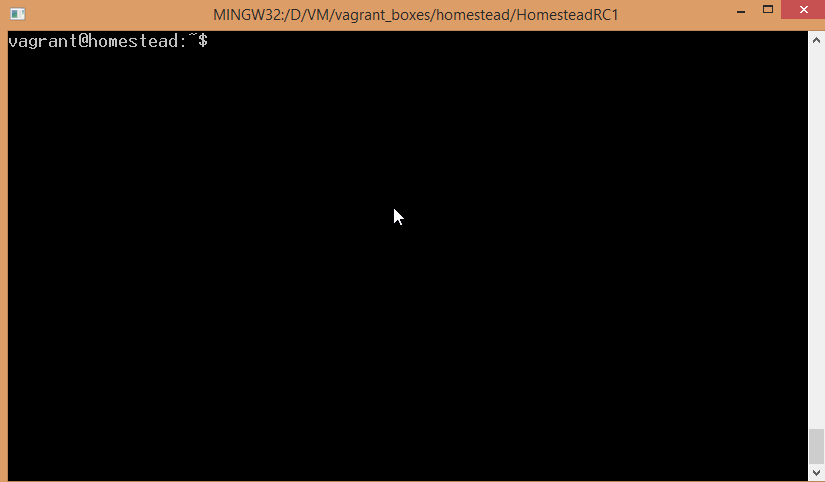
When writing efficient JavaScript tests, using a testing framework is the best place to start. Testing frameworks provide developers with a suite of tools and utilities that make writing and running tests more effortless and streamlined. Some popular JavaScript testing frameworks include Jasmine, Mocha, and Jest. These frameworks provide a range of features and tools, such as assertion libraries, test runners, and mocking tools, to make writing tests more efficient and effective.
One of the foremost benefits of using a testing framework is that it saves time and effort. Rather than writing every aspect of the test from scratch, the framework provides pre-built tools and utilities that can be used to write tests more quickly and easily. This makes testing a more efficient process, allowing developers to focus on writing code rather than writing tests.
Additionally, testing frameworks are designed to work seamlessly with JavaScript, making integrating testing into the development process more manageable. For example, many testing frameworks allow developers to run tests automatically when code changes are made, ensuring that any issues are caught early in the development process.
Using a testing framework also ensures consistency across tests. When using a framework, all tests will follow a similar structure and format, making it easier to read and understand the tests. This consistency makes it easier to maintain the codebase and ensure that tests are effective in catching bugs and issues.
Finally, using a testing framework can help with documentation. Tests written using a framework can serve as a form of documentation, showing how the code is intended to be used and what its expected behavior is. This documentation can be invaluable for future developers working on the codebase, as it provides a clear understanding of the code and its intended use.
2. Use Code Coverage Tools
These tools work by analyzing the codebase and identifying which parts of the code are being executed during testing. They then provide a report that shows the percentage of code covered by tests, highlighting areas of the codebase that may need more testing.
Using code coverage tools can help ensure that all parts of the codebase are tested, reducing the likelihood of bugs and issues. Additionally, code coverage tools can help identify areas of the codebase that are not being tested, allowing developers to write more tests to cover those areas. Jest provides built-in support for code coverage reporting, making it easy to generate reports showing the percentage of code covered by tests.
3. Use Setup and Teardown Functions
Using setup functions, you can ensure that each test starts with a clean slate and has a key to the resources it needs to run. Setup functions are used to set up the test environment before each test is run. This can include creating mock objects, setting up test data, or establishing connections to external resources.
Teardown functions are used to clean up the test environment after each test has been completed. This can include closing connections, removing temporary files, or deleting test data. By using teardown functions, you can ensure that the test environment is reset to its original state, making it ready for the next test.
Jest provides support for setup and teardown functions, making it easy to write tests that are self-contained and isolated from each other. With these functions, you can write tests that are more reliable and easier to maintain, as they do not rely on external resources that may change over time.
4. Use Snapshot Testing
This involves saving the expected output of a function or component and comparing it to the actual output during testing. If there is a difference between the expected and actual output, the test will fail, indicating a change in the code.
Jest provides built-in support for snapshot testing, making it easy to write snapshot tests for your code. When you run a snapshot test for the first time, Jest saves a snapshot of the expected output. When the same test is rerun, Jest compares the actual output to the saved snapshot. If the output matches, the test passes. If there are differences, the test fails, and Jest provides a difference to help identify the changes.
Snapshot testing can be beneficial for testing user interfaces, where changes may not be immediately apparent. By taking snapshots of the UI components and comparing them during testing, you can quickly identify unexpected changes in your code.
5. Use Descriptive Test Names
When writing tests, it’s essential to use descriptive names that convey the test’s purpose. This makes it easier to identify which tests are failing and why. For example, if you are testing a function that adds two numbers, a good test name would be “should return the sum of two numbers.” This name conveys what the test is testing, making it easier for anyone reading the test results to understand the purpose of the test.
Additionally, using active language in your test names can make them even more descriptive. The active language emphasizes what the code should do rather than simply describing it. For example, instead of “test if the function returns true,” you could write “should return true.” This makes the purpose of the test more explicit and easier to understand.
6. Write Small, Focused Tests
Small tests are more accessible to write and maintain than large, complex tests. Each test should focus on a specific feature or behavior of the code being tested. This makes it easier to isolate bugs and fix them quickly, reducing the time and effort required to identify and resolve issues.
Focusing on small tests also makes it easier to refactor code without breaking existing tests. By breaking the code into smaller, more focused tests, developers can ensure that each test only verifies a single code behavior. This makes it easier to identify which tests will be affected by a code change and update them accordingly.
By running small tests in parallel, developers can reduce the overall time required to run the test suite and receive feedback on their changes quickly. This allows them to make changes and iterate on their code more quickly, leading to faster development cycles. Small tests are also more resilient to changes in the codebase and are easier to run in parallel.
7. Use Mocks and Stubs
Mocks are used to simulate external dependencies, such as network calls or database queries. In contrast, stubs replace specific functions or methods with a test implementation. Using mocks and stubs can help reduce the complexity of tests and make them more focused.
Mocking external dependencies can be particularly useful when testing code that relies on external resources, such as APIs or databases. By simulating these dependencies with mocks, developers can ensure that tests are run consistently and do not rely on external resources that may be unreliable or slow. Additionally, mocking can make tests more deterministic, allowing developers to control the responses and behavior of external dependencies.
Stubs, on the other hand, are helpful when testing code that relies on specific functions or methods. By replacing these functions or methods with a test implementation, developers can ensure that tests are not affected by changes made to the original code. Stubs can also be used to simplify tests, as they allow developers to focus on the specific behavior being tested rather than the behavior of the dependent function or method.
8. Use Parameterized Tests
It involves running the same test with multiple inputs rather than writing a separate test for each input. This can help downsize code duplication and make tests more efficient.
When using parameterized tests, developers can define an array of inputs and expected outputs for a specific test. The test code then runs the same set of assertions on each input in the array. This approach can reduce the number of tests that need to be written, as it allows developers to test a wide range of inputs with a single test.
Parameterized tests can also make it easier to maintain tests over time. When new input values need to be added or removed, only the input array needs to be updated rather than modifying multiple tests. This can save time and reduce the likelihood of errors in the testing process.
9. Use Test-Driven Development (TDD)
Test-Driven Development (TDD) has become a popular development approach in JavaScript development. This approach emphasizes writing tests before writing code. The idea is to write a test that fails before writing any code and then write just enough code to make the test pass. This approach ensures that the code being written meets the test requirements and that the tests verify any changes made to the code.
TDD can also help improve code quality and reduce the number of bugs in the codebase. By writing tests first, developers can ensure that the code they write is thoroughly tested and meets the project’s requirements. Additionally, TDD can help ensure that code is more modular and easier to maintain, as it encourages developers to write code in smaller, more manageable chunks.
TDD even ensures that new code does not break existing functionality. When changes are made to the codebase, developers can run the tests to ensure that any changes made do not break any existing functionality. This can reduce the number of bugs in the codebase and ensure that the codebase remains stable over time.
10. Use Continuous Integration (CI)
Using CI with JavaScript tests can help ensure that the codebase is always working. CI tools such as Jenkins, Travis CI, and CircleCI can automatically run JavaScript tests whenever new code is added to the repository. This ensures that any issues or bugs are caught early on, reducing the time and effort required to fix them. By detecting issues early, developers can ensure that the codebase is always stable, even during active development.
Using CI with JavaScript tests also helps ensure that tests are run consistently across different environments. This can be particularly useful when developing for different platforms, such as web and mobile. By running tests automatically on different environments, developers can ensure their code works consistently across platforms.
Conclusion
The need for reliable and efficient code continues to grow as the world becomes more reliant on technology. To keep up with this demand, developers must continue to adopt new techniques and best practices for automation testing.
We expect to see even more advanced testing techniques and frameworks in JavaScript development. As the industry evolves, staying up-to-date with all the latest tools and market trends is crucial to ensure that our code remains efficient, reliable, and bug-free. If you’re looking for a reliable cloud-based platform to perform efficient JavaScript testing with Jest, LambdaTest is worth exploring.
To introduce, LambdaTest is a unified intelligent digital experience testing platform that enables developers and testers to test their web and mobile applications over more than 3000+ browsers and OS configurations. LambdaTest also provides a range of features to optimize your JavaScript testing, such as screenshot testing, video recording, and network logs analysis. With these features, you can quickly identify and troubleshoot issues with your JavaScript tests and improve the overall quality of your application.